Table of Contents
Hello again, I welcome each and everyone of you.
Today we will go on another Journey to make a react native countdown timer example using momentJs.
And as usual I will try to make it a bit good looking. So stay with e for a while and let’s get started.
Here is the final result
Environment setup
To get started just create a new react native project, on this project I will use Expo Cli.
so the command is.
$ expo init projectname
After the react native setup is finished, your code and screen will look similar to this.
import React from 'react';
import { StyleSheet, Text, View } from 'react-native';
export default class App extends React.Component {
render(){
return (
<View style={styles.container}>
<Text>Open up App.js to start working on your app!</Text>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});

MomentJs
To create and manipulate dates, we are gonna use time parse and manipulate library called MomentJs.
MomentJs has it all when it comes to date and time manipulates.
It literally can do anything you can think of when it comes to time. From simple additions and subtractions to relative date display, such as 6 days ago etc.
So, we need to install it first
$ yarn add moment
Then import it in your project
import moment from "moment"
React Native Countdown Timer Concept
Like I said, momentjs will help us manipulate time in our app, but it doesn’t came a timer function, so we’ll make one.
The idea here, is to save the current time, then add to it the duration of the event we want to count down from, as an example adding 3 days to the current time.
Then, we will write a function to subtract a second on ever second that passes, thus we can get the days, hours, minutes and seconds of the duration to display on our screen.
Let’s get started
initial state
Let’s prepare our state with our initial values
state={
eventDate:moment.duration().add({days:1,hours:3,minutes:40,seconds:50}), // add 9 full days, 3 hours, 40 minutes and 50 seconds
days:0,
hours:0,
mins:0,
secs:0
}
On the eventDate field, we are going to save the current moment as a duration using monentJs’ duration function.
then add to it the event desirable duration to it as on object, Like you seen on code.
You can add more than just days, hours, minutes and seconds, go up to weeks, months and years.
Next, we will save the list of days, hours, minutes and seconds on display on our UI.
notice that these are the variables to change on every second that passes.
Update Time Function
We need to create a function to manipulate our duration event.
updateTimer=()=>{
const x = setInterval(()=>{
let { eventDate} = this.state
if(eventDate <=0){
clearInterval(x)
}else {
eventDate = eventDate.subtract(1,"s")
const days = eventDate.days()
const hours = eventDate.hours()
const mins = eventDate.minutes()
const secs = eventDate.seconds()
this.setState({
days,
hours,
mins,
secs,
eventDate
})
}
},1000)
}
The function is based on an interval of one seconds, which will run our code every second.
The function then checks wether the eventDuration is equal to or smaller than 0, since the duration is saved in milliseconds.
And its basically the time different between our the current time and the time we gave it, its value being 0 means the time has reached the end, if it’s greater than 0
However meaning the time is steal going, and of course if it goes below 0, it’s value becomes negative and we do not want that to appear on our UI.
Hence, we clear the interval when the timer reaches a 0 or negative value.
As long as the timer is positive, we save the days, hours, minutes, and seconds of the duration, and we subtract 1 seconds from the event timer.
Since a minute has passed and we need to countdown timer to update.
Then, we update the state to cause a re-render of the UI.
After its fully created, let’s add to the the componentDidMount lifecycle method.
componentDidMount(){
this.updateTimer()
}
UI Update
Let’s change the Text View method of the app to display the current timer
render(){
const { days, hours, mins, secs } = this.state
return (
<View style={styles.container}>
<Text>{`${days} : ${hours} : ${mins} : ${secs}`}</Text>
</View>
);
}
Now our app looks, something like this.

Whoa, Not bad. If your concern was to create a react native countdown timer, there you have it, you have reached your desire.
But, I wont stop here, I am going to add some style to it.
Add Background Image
First let’s get rid of that senseless white background.
I have done some quick research to find an ideal background image and made it into this one.
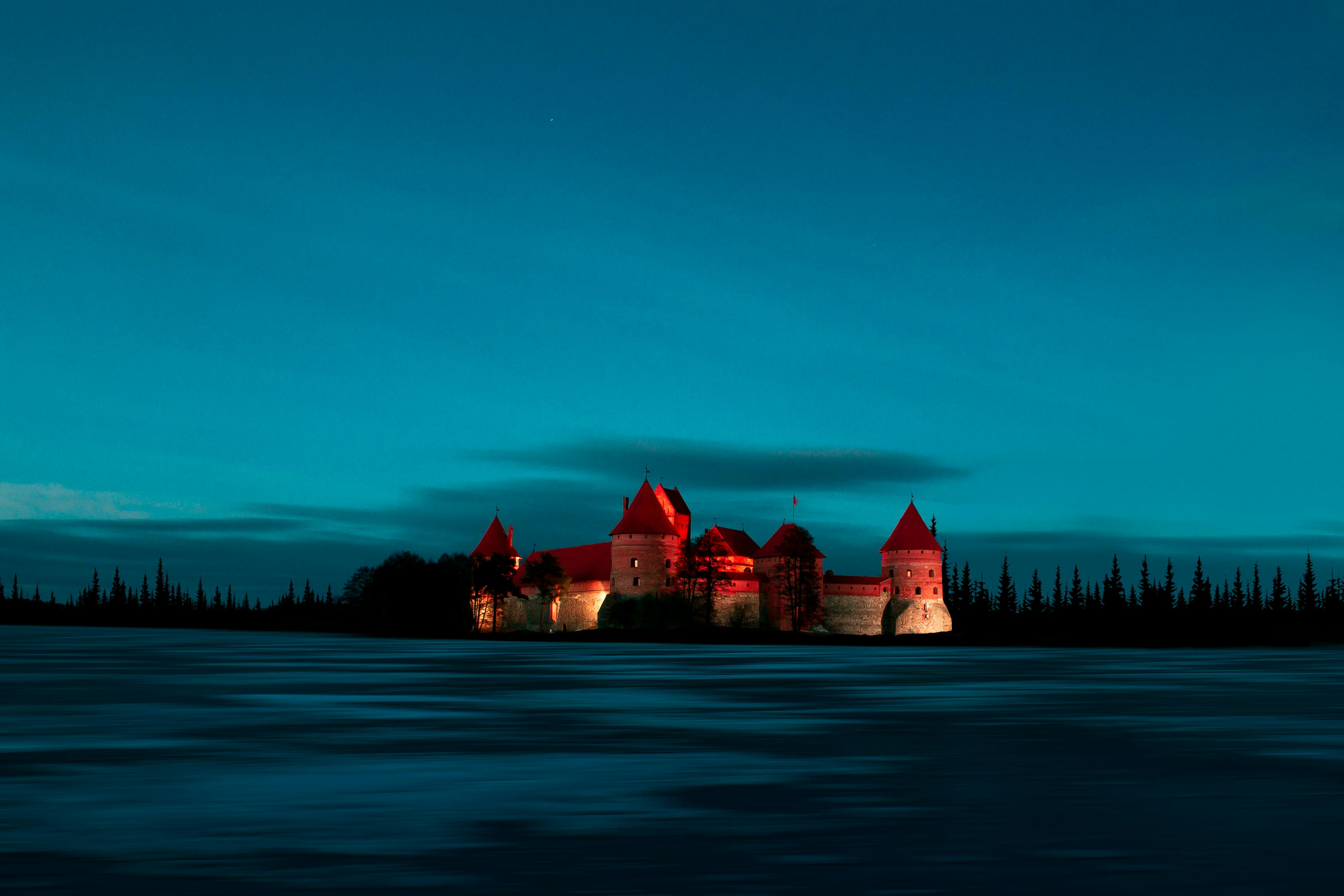
Using React Native ImageBackground Component
Back when react native first came it it was a real work ti put a background image into a view.
you needed to rely heavily on CSS to achieve such thing.
Lately React native added an ImageBackground component just for this, and it’s really helpful.
So first you need to import it, lts a part of the react native library.
so no need to install anything. just import it.
Then, I am going to replace the root View of our app to an ImageBackground component.
the only thing that we will need to add is the path to the image.
So download the image and add it to your assets folder.
The render method now looks like this.
<ImageBackground source={require("./assets/bg.jpg")} style={styles.container}>
<Text style={{fontWeight:"bold",fontSize:50}}>
{`${days} : ${hours} : ${mins} : ${secs}`}
</Text>
</ImageBackground>
One more thing I have added, is a quick styling to the Text View as well.
which is just a bold font weight property and a font size of 50, to make the text larger and thicker.

Next, I am to add one more Text view to the screen saying Coming soon.
give it a reddish color color, bold style above the current timer text View.
I will also add some margin to the timer text to slide it top a bit.
and this is our final look.

Thank you so much for your time, I hope you enjoyed my article. Stay tuned for more.
If you would like to try this app on expo client, I have published the project on expo.io project .
I have also made a github repository for the project.
Recommended Articles
React Native Flatlist Example
React Native Camera Expo Example
React Native Accessibilityinfo API
Thanks for the example, nice one and easy to understand